How To Control L298n Motor Drive With Arduino
How to control DC motor with L298N driver and Arduino.
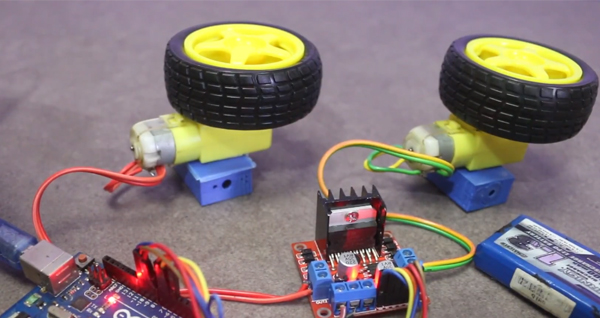
Hi, In this article you will learn, how you can control two DC motors with Arduino using L298N motor driver module
L298N motor driver module.
L298N H-bridge motor driver module is use to control two DC motor or a single bipolor stepper motor. By using this module you can control direction and speed of DC motors. This module support 5 to 35 volt DC.
In this module also have onboard 5v regulator. so if you are using 6 to 12v power supply then you have also have 5 volt output from the board.
Control DC motors with Arduino
When you want to drive DC motor with Arduino, you needs some sort of motor driver module which can run motors and control the direction and speed of the motors. L298N driver module is compact and best for this. In this post I have attached step by step demonstration video tutorial to control speed and diraction of DC motors with motor driver module, manually as well as with Arduino.
Video tutorial for controlling DC motors with driver module.
Arduino code for L298N motor driver module.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
| // connect motor controller pins to Arduino digital pins // motor one int enA = 10; int in1 = 9; int in2 = 8; // motor two int enB = 5; int in3 = 7; int in4 = 6; void setup() { // set all the motor control pins to outputs pinMode(enA, OUTPUT); pinMode(enB, OUTPUT); pinMode(in1, OUTPUT); pinMode(in2, OUTPUT); pinMode(in3, OUTPUT); pinMode(in4, OUTPUT); } void demoOne() { // this function will run the motors in both directions at a fixed speed // turn on motor A digitalWrite(in1, HIGH); digitalWrite(in2, LOW); // set speed to 200 out of possible range 0~255 analogWrite(enA, 200); // turn on motor B digitalWrite(in3, HIGH); digitalWrite(in4, LOW); // set speed to 200 out of possible range 0~255 analogWrite(enB, 200); delay(2000); // now change motor directions digitalWrite(in1, LOW); digitalWrite(in2, HIGH); digitalWrite(in3, LOW); digitalWrite(in4, HIGH); delay(2000); // now turn off motors digitalWrite(in1, LOW); digitalWrite(in2, LOW); digitalWrite(in3, LOW); digitalWrite(in4, LOW); } void demoTwo() { // this function will run the motors across the range of possible speeds // note that maximum speed is determined by the motor itself and the operating voltage // the PWM values sent by analogWrite() are fractions of the maximum speed possible // by your hardware // turn on motors digitalWrite(in1, LOW); digitalWrite(in2, HIGH); digitalWrite(in3, LOW); digitalWrite(in4, HIGH); // accelerate from zero to maximum speed for ( int i = 0; i < 256; i++) { analogWrite(enA, i); analogWrite(enB, i); delay(20); } // decelerate from maximum speed to zero for ( int i = 255; i > 0; --i) { analogWrite(enA, i); analogWrite(enB, i); delay(20); } // now turn off motors digitalWrite(in1, LOW); digitalWrite(in2, LOW); digitalWrite(in3, LOW); digitalWrite(in4, LOW); } void loop() { demoOne(); delay(1000); demoTwo(); delay(1000); } |
No comments